Microsoft is back at it: KB2952664 and KB2976978 rear their ugly heads again
2 min. read
Published on
Read our disclosure page to find out how can you help Windows Report sustain the editorial team Read more
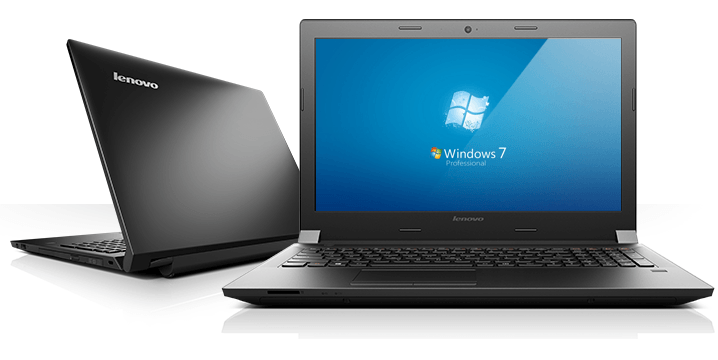
Last month, we reported that Microsoft released the infamous Windows 7, 8.1 KB2952664 and KB2976978 updates again. If you thought that you would’t have to deal with these two updates, think again because they’re back.
Updates KB2952664 and KB2976978 are perhaps the most mysterious Windows updates. Many users suggest that they are part of Microsoft’s spy toolkit and refuse to install them on their computers.
On the other hand, Microsoft claims the two updates only evaluate the compatibility status of the Windows ecosystem. In other words, Microsoft relies on KB2952664 and KB2976978 to ensure application and device compatibility for all Windows updates. However, the company hasn’t yet offered a clear answer regarding the spying accusations made by many users.
KB2952664 and KB2976978 — an IT Bermuda triangle
On KB2952664′ s support page, Microsoft uses the same description it has always used:
This update performs diagnostics on the Windows systems that participate in the Windows Customer Experience Improvement Program. The diagnostics evaluate the compatibility status of the Windows ecosystem, and help Microsoft to ensure application and device compatibility for all updates to Windows. There is no GWX or upgrade functionality contained in this update.
In other words, the mystery of the two updates remains intact. Many users claim that KB2952664 and KB2976978 run a task called DoScheduledTelemetryRun. Following the telemetry revelations from last year, they suggest that the two updates have covert purposes.
However, this suggestion remains a simple hypothesis as no clear proof has been found to fully support it. If you blocked these two updates last month, you’ll need to block them again now that Microsoft reissued them.
Have you installed KB2952664 or KB2976978 on your computer? Did you notice anything particular about the March edition of these updates?
RELATED STORIES YOU NEED TO CHECK OUT:
- Windows 10 Creators Update’s privacy settings raise new concerns
- Windows 10 privacy gets major changes to win over suspicious users
- The 7 best proxy tools for Windows 10 to protect your privacy